[Python] 파이썬으로 만드는 마인크래프트 스타일 블록 게임
pygame 하나면 충분하다!
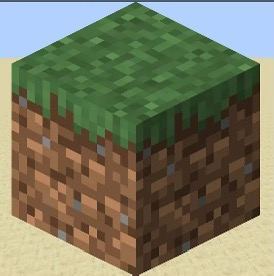
1. 왜 이런 게임을 만들게 되었냐면요…
음… 사실 나는 마인크래프트를 진짜 많이 하진 않았는데, 가끔 유튜브 보다가 블럭 쌓는 거 보면 뭔가 뿌듯해지더라고요? ^^ 그래서 “어? 나도 파이썬으로 이런 거 간단하게 만들어볼 수 있지 않을까?” 하는 마음에 도전해봤어요.
완전 똑같이 만들 순 없겠지만, 최소한
“블럭을 깔고, 없애고, 이동할 수 있는” 정도의 기본은 구현해보자!
이렇게 목표를 잡고 코딩을 시작했습니다.
⸻
2. 개발 환경
• Python 3.9+
• pygame (pip로 설치 가능해요)
pip install pygame
그냥 이거 하나면 끝나요!
pygame은 2D 게임 만들기 딱 좋더라고요 ^^
⸻
3. 게임의 규칙과 기능
• WASD 키로 캐릭터 이동
• 마우스 왼쪽 클릭: 블럭 설치
• 마우스 오른쪽 클릭: 블럭 제거
• 중력 적용 (떨어지는 느낌 구현)
• 블럭은 여러 개 깔 수 있음!
⸻
4. 전체 코드 (하나의 파일!)
이 코드는 minecraft_like.py 같은 이름으로 저장하면 됩니다!
import pygame
import sys
# 기본 설정
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
TILE_SIZE = 40
GRAVITY = 1
JUMP_POWER = -15
# 색상 정의
WHITE = (255, 255, 255)
GRAY = (100, 100, 100)
BLUE = (0, 100, 255)
GREEN = (0, 255, 0)
# 초기화
pygame.init()
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("마인크래프트st 블록 게임 ^^")
clock = pygame.time.Clock()
# 블럭 저장용 딕셔너리
blocks = {}
# 플레이어 정의
player = pygame.Rect(100, 100, TILE_SIZE, TILE_SIZE)
player_y_velocity = 0
on_ground = False
def get_tile(pos):
return (pos[0] // TILE_SIZE, pos[1] // TILE_SIZE)
def draw_blocks():
for (x, y), color in blocks.items():
pygame.draw.rect(screen, color, pygame.Rect(x*TILE_SIZE, y*TILE_SIZE, TILE_SIZE, TILE_SIZE))
while True:
screen.fill(WHITE)
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 마우스 클릭으로 블럭 추가/삭제
if event.type == pygame.MOUSEBUTTONDOWN:
mouse_tile = get_tile(pygame.mouse.get_pos())
if event.button == 1: # 왼쪽 클릭
blocks[mouse_tile] = GRAY
elif event.button == 3: # 오른쪽 클릭
if mouse_tile in blocks:
del blocks[mouse_tile]
keys = pygame.key.get_pressed()
dx = 0
if keys[pygame.K_a]:
dx = -5
if keys[pygame.K_d]:
dx = 5
if keys[pygame.K_SPACE] and on_ground:
player_y_velocity = JUMP_POWER
# 중력 적용
player_y_velocity += GRAVITY
dy = player_y_velocity
# 이동
player.x += dx
player.y += dy
# 충돌 처리
on_ground = False
for (bx, by), color in blocks.items():
block_rect = pygame.Rect(bx*TILE_SIZE, by*TILE_SIZE, TILE_SIZE, TILE_SIZE)
if player.colliderect(block_rect):
if dy > 0:
player.bottom = block_rect.top
player_y_velocity = 0
on_ground = True
elif dy < 0:
player.top = block_rect.bottom
player_y_velocity = 0
# 바닥 체크
if player.bottom > SCREEN_HEIGHT:
player.bottom = SCREEN_HEIGHT
player_y_velocity = 0
on_ground = True
# 블럭 그리기
draw_blocks()
# 플레이어 그리기
pygame.draw.rect(screen, BLUE, player)
pygame.display.flip()
clock.tick(60)
⸻
5. 실행 결과 ^^!
실행하면 이런 화면이 나올 거예요:
• 파란 캐릭터가 움직이고
• 마우스로 블럭을 깔 수 있어요 ^^
• 뛰어다니면서 쌓고 깨고… 재밌어요 -_-
⸻
6. 마무리하며…
물론 이건 진짜 마인크래프트에 비하면 아주 귀여운 수준이죠.
하지만 파이썬으로 이런 게임을 하나의 파일로 직접 만들었다는 게 더 뿌듯해요.
여기서 더 발전시키고 싶다면?
• 블럭 종류 추가하기 (나무, 돌 등)
• 월드 저장/불러오기
• 점프 애니메이션 넣기
• 배경 음악 넣기 (pygame.mixer로 가능!)
도전해보세요! 나중에 멀티플레이도 만들 수 있…을지도…? ^^;;